blogs
Understanding and Mitigating Memory Leaks in Flutter Applications
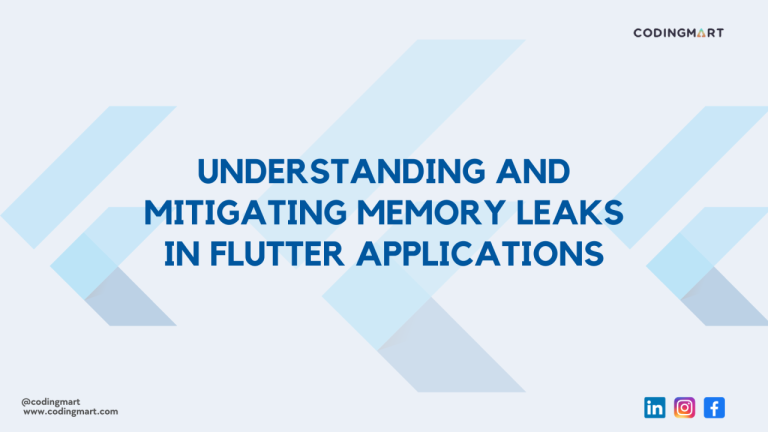
We unravel the challenges posed by memory leaks in Flutter applications and explore effective strategies to detect, address, and prevent these memory hiccups. Join us as we navigate through common causes, detection tools, and preventive measures, empowering you to optimize your Flutter app’s performance and deliver an impeccable user experience.
Memory leaks in Flutter applications occur when the program holds unnecessary memory, leading to excessive memory consumption. This can result from unused objects lingering in memory due to caching, improper disposal, or neglecting to remove listeners, ultimately impacting performance and user experience.
Mishandling streams, integral for managing asynchronous events in Flutter, can also contribute to memory leaks if stream subscriptions are not correctly dropped, causing the stream to persist in the background and consume memory. Additionally, loading large images and videos without proper release can exacerbate memory leaks in Flutter applications.
Common Causes of Memory Leaks in Flutter:
- Unused Objects: Objects, such as unclosed streams, can persist in memory, causing leaks when not properly managed. This includes instances where streams are created but not closed, leading to lingering references.
- Improper Use of Streams: Misusing streams in Flutter for handling asynchronous events can result in memory leaks. Failure to cancel stream subscriptions appropriately allows streams to persist in the background, consuming memory.
- Global Variables: Storing references to objects or widgets as global variables prevents the garbage collector from functioning correctly, necessitating memory deallocation to prevent leaks.
- Large Images and Videos: Loading substantial images and videos without releasing them when no longer needed can lead to significant memory leaks, as these files consume a considerable amount of memory.
- Widget Trees: Incorrectly placing widgets on the widget tree, especially when using stateful widgets, can also be a source of memory leaks in Flutter.
Detecting and Addressing Memory Leaks in Flutter:
– Flutter DevTools: Utilize Flutter DevTools, a performance and debugging tool, to examine your application’s memory usage. Access it through the command: `flutter pub global run devtools`.
– Heap Snapshots: Take heap snapshots to analyze memory utilization and identify objects not being garbage collected as expected.
– Code Analysis: Review your code, paying attention to proper disposal of objects, with a focus on controllers, a common cause of memory leaks in Flutter.
Preventing Memory Leaks in Flutter:
- Dispose of Objects: Discard unnecessary objects using the `dispose` method in Stateful Widgets to free up resources.
- Stream Management: Cancel stream subscriptions when not needed to prevent streams from persisting in the background and consuming memory.
- Efficient Media Handling: Use techniques like the `flutter_cache_manager` package to efficiently load and cache images and videos, reducing memory consumption.
- Profiling Tools: Leverage tools like Flutter DevTools to identify and fix memory leaks by analyzing your application’s memory utilization.
- Thread Safety: Avoid placing Flutter object references in background threads. Instead, use weak references or isolate communication mechanisms to prevent strong references and guard against memory leaks.
Conclusion:
 Crafting seamless Flutter applications involves mastering the art of memory management. As we wrap up this, equip yourself with the knowledge to identify and mitigate memory leaks effectively. Let’s build applications that not only dazzle users with their features but also run smoothly under the hood. Until next time, happy coding!