blogs
Leveraging Promisification in Modern JavaScript Development
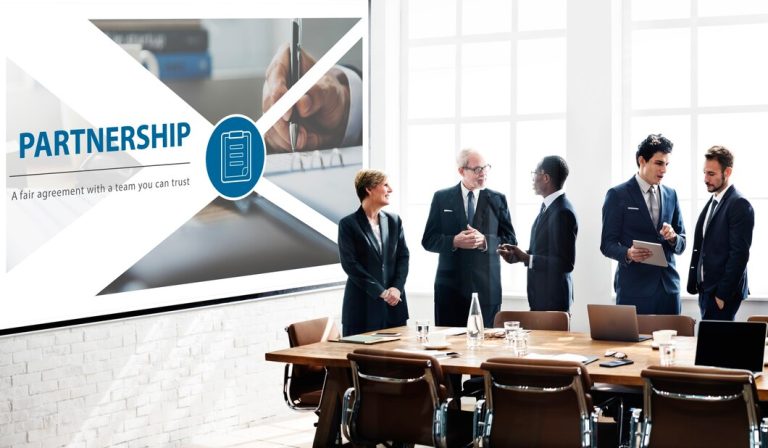
In the ever-evolving landscape of software development, efficiency and readability stand as paramount virtues. One methodology that has gained prominence in recent times is “Promisification.” This paradigm shift leverages the power of JavaScript Promises, transforming asynchronous code into a more concise and readable form. Let’s delve into the essence of promisification and understand how it can elevate your coding experience.
What are callback and promise?
The callback is a function that is passed as a parameter to another function. And this function will be called inside the function.
A promise is an object which is used to handle asynchronous operations. It is used to handle multiple asynchronous operations which may have a dependency on each other.
Why do we need a callback and promise?
Callback and promise are used to handle asynchronous operations. An asynchronous operation is an operation that is not executed immediately. It is executed after some time. For example, if you are making a request to the server to get some data, then it will take some time to get a response from the server. So, we need to wait for the response. If we are making multiple requests to the server, then we need to wait for all the responses. So, we need to handle asynchronous operations.
What is Promisification and Why do we need them?
Promisification is the process of converting traditional callback-based asynchronous code into a Promise-based structure. This not only enhances the readability of the code but also simplifies error handling and promotes a more modular approach to development.
One significant advantage of promisification is evident in its ability to streamline code execution. By encapsulating asynchronous operations within Promises, developers can chain multiple asynchronous calls effortlessly, creating a sequential flow that mirrors the natural progression of the program.
Moreover, error handling becomes more intuitive with Promises. The traditional callback approach often led to “callback hell,” making it challenging to manage errors effectively. Promises address this by providing a cleaner syntax for handling both success and error conditions through the `.then()` and `.catch()` methods.
Example:
Consider the following example of promisification in a Node.js environment:
const fs = require(‘fs’);
function readFileAsync(path) {
  return new Promise((resolve, reject) => {
    fs.readFile(path, ‘utf8’, (err, data) => {
      if (err) {
        reject(err);
      } else {
        resolve(data);
      }
    });
  });
}
// Using the promisified function
readFileAsync(‘example.txt’)
  .then(data => console.log(data))
  .catch(error => console.error(error));
In this example, the `readFileAsync` function promisifies the `fs.readFile` operation, allowing for a more elegant and manageable way to handle file reading.
Let’s consider the conventions used in Node.js-style callback based functions:
- The callback is the last argument of the function
- The error (if any) is always the first argument passed to the callback
- Any return value is passed after the error to the callback
Based on these rules, we can easily create a generic function that promisifies a Node.js-style callback-based function. Let’s see what this function looks like:
function promisify (callbackBasedApi) {
 return function promisified (…args) {
   return new Promise((resolve, reject) => {
     const newArgs = [
       …args,
       function (err, result) {
         if(err) {
           return reject(err)
         }
         resolve(result)
       }
     ]
     callbackBasedApi(…newArgs)
   })
 }
}
The preceding function returns another function called promisified() , which represents the promisified version of the callbackBasedApi given as the input. This is how it works:
- The promisified() function creates a new Promise using the Promise constructor and immediately returns it to the caller.
- In the function passed to the Promise constructor, we make sure to pass to callbackBasedApi a special callback. Since we know that the callback always comes last, we simply append it to the arguments list ( args ) provided to the promisified() function. In the special callback, if we receive an error, we immediately reject the Promise ; otherwise, we resolve it with the given result .
- Finally, we simply invoke callbackBasedApi with the list of arguments we have built.
Promisification emerges as a powerful technique, bringing clarity and simplicity to asynchronous JavaScript code. Its impact goes beyond mere syntax refinement, extending to improved error handling, modularization, and overall code maintainability. As we navigate the dynamic landscape of modern development, embracing promisification can be a transformative step towards crafting cleaner, more efficient code.
This methodology not only aligns with the trajectory of JavaScript evolution but also fosters a coding environment where readability and maintainability thrive. Consider integrating promisification into your development workflow to unlock a new realm of possibilities in asynchronous programming.