blogs
Choosing Between Async/Await and Promises in JavaScript | Guide
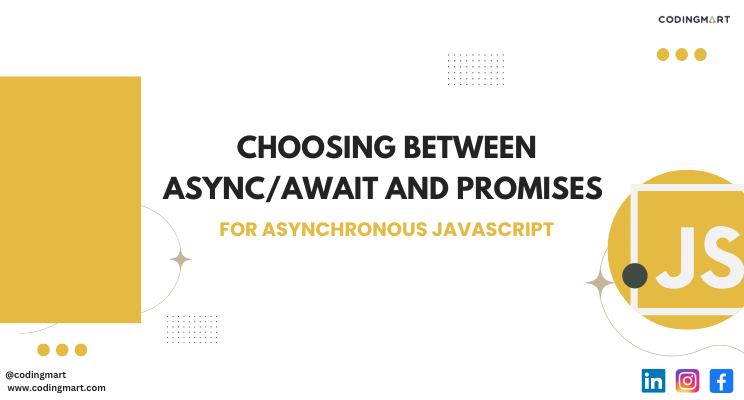
In the realm of asynchronous JavaScript, developers have various options such as callbacks, generators, promises, and the newer async/await. While callbacks are considered the older approach, we’ll focus on the debate between promises and async/await, keeping in mind the goal of writing cleaner and more readable code.
Async/Await: The Synchronous-Like Asynchronous Code:
Introduced in ECMAScript 2017, async/await revolutionized asynchronous code by introducing a more synchronous-looking syntax. The async keyword marks a function as asynchronous, and the await keyword allows for pausing execution until a promise is resolved. Let’s dive into a more elaborate example:
const fetchData = async () => {
 try {
 const data = await fetchDataFromAPI();
 const processedData = await processData(data);
 console.log(processedData);
 } catch (error) {
 console.error(“Error:”, error);
 }
};
fetchData();
In this example, the code reads sequentially, making it easier to follow the flow of asynchronous operations. The try/catch block enhances error handling, providing a clean and concise structure.
Promises: The Foundation of Asynchronous JavaScript:
Promises, introduced in 2012, laid the foundation for structured and manageable asynchronous code. They operate based on three states: pending, resolved, and rejected. The then() and catch() methods allow developers to handle successful and failed outcomes, respectively. A more detailed example demonstrates the fundamental principles:
const fetchData = new Promise((resolve, reject) => {
 fetchDataFromAPI()
 .then((data) => processData(data))
 .then((processedData) => resolve(processedData))
 .catch((error) => reject(error));
});
fetchData
 .then((result) => console.log(result))
 .catch((error) => console.error(“Error:”, error));
While promises offer clarity and are an integral part of JavaScript, chaining them can sometimes lead to nested structures that may become challenging to decipher.
Async/Await vs. Promises: A Comparative Analysis:
In the eternal debate of async/await vs. Promises, several aspects come into play:
- Readability and Conciseness: Async/await tends to produce more readable and concise code, making it easier to understand the logic and flow of asynchronous operations.
- Error Handling: The try/catch structure in async/await simplifies error handling, providing a more elegant alternative to promise chaining and catch().
- Exclusive Use of Await: The await keyword can only be used inside functions marked with async, preventing its misuse and providing a clear indication of asynchronous operations.
- Sequential Execution: Async/await fosters a more sequential and declarative style, making the code resemble synchronous operations and facilitating easier comprehension.
Going Beyond Syntax: A Deeper Dive into Readability:
In the pursuit of clean code, readability extends beyond syntactical choices. Naming conventions, code structure, and declarative syntax significantly impact how comprehensible a codebase is. Async/await, with its sequential nature, often translates into code that reads like a natural language sentence.
Promises provide a clear structure for handling asynchronous tasks and can lead to readable code, especially when compared to the older callback-based approaches. The .then() and .catch() chaining allows for a more organized flow of asynchronous operations, making it easier to follow the logic.
However, in certain scenarios where multiple asynchronous operations are involved, and chaining becomes extensive, code written with Promises might become nested and complex. This situation is often referred to as “callback hell” or “Promise hell,” and it can affect code readability.
This is where async/await shines. It introduces a more synchronous-style syntax, making the code read like a series of sequential steps, which many developers find more readable and easier to understand. The async/await syntax simplifies error handling with the use of try/catch blocks, contributing to code that resembles natural language.
Elevating Code Quality Through Informed Choices:
Asynchronous JavaScript affords developers the flexibility to choose between async/await and Promises. The decision ultimately hinges on project requirements, team preferences, and the commitment to producing high-quality, maintainable code. By understanding the nuances of both approaches, developers can make informed decisions that align with their coding principles. Whether it’s the synchronous allure of async/await or the robust foundation of Promises, let the choice reflect your dedication to writing exceptional JavaScript. Happy coding!